https://school.programmers.co.kr/learn/courses/30/lessons/77485
프로그래머스
코드 중심의 개발자 채용. 스택 기반의 포지션 매칭. 프로그래머스의 개발자 맞춤형 프로필을 등록하고, 나와 기술 궁합이 잘 맞는 기업들을 매칭 받으세요.
programmers.co.kr
Lv 2
구현 방법
조건은
queries의 행의 개수(회전의 개수)는 1 이상 10,000 이하입니다.
1 ≤ x1 < x2 ≤ rows, 1 ≤ y1 < y2 ≤ columns입니다.
이므로 그냥 이중 포문으로 돌리면 된다.
시계 방향으로 숫자가 하나씩 밀리는 거라서, 반시계 방향으로 숫자를 하나씩 바꾸면 된다.
이런식으로 하면 맨 처음의 숫자만 따로 임시로 기억해 놓으면, 나머지 숫자는 임시로 기억안해도 돼서, 구현이 편해진다.
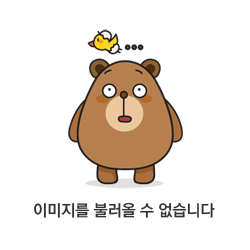
주의할 점은, 마지막에 기억한 숫자 업데이트 해줘야 하고, 문제에서 "i 행 j 열에 있는 숫자는 ((i-1) x columns + j)입니다." 라고 했는데 이건 배열 시작을 (1,1)로 했을때 기준이라서 (i * columns + j + 1) 이렇게 바꿔야 한다.
코드
class Solution {
public int[] solution(int rows, int columns, int[][] queries) {
int[] answer = new int[queries.length];
int[][] plate = new int[rows][columns];
for(int i = 0; i < rows; i++) {
for (int j = 0; j < columns; j++) {
plate[i][j] = (i * columns + j + 1);
}
}
for (int i = 0; i < queries.length; i++) {
int startRow = queries[i][0] - 1;
int startCol = queries[i][1] - 1;
int endRow = queries[i][2] - 1;
int endCol = queries[i][3] - 1;
int firstNum = plate[startRow][endCol];
int min = firstNum;
//오른쪽
for(int j = endCol; j > startCol; j--) {
plate[startRow][j] = plate[startRow][j - 1];
min = Math.min(min, plate[startRow][j]);
}
//위
for(int j = startRow; j < endRow; j++) {
plate[j][startCol] = plate[j + 1] [startCol];
min = Math.min(min, plate[j][startCol]);
}
//왼쪽
for(int j = startCol; j < endCol; j++) {
plate[endRow][j] = plate[endRow][j + 1];
min = Math.min(min, plate[endRow][j]);
}
//아래
for(int j = endRow; j > startRow; j--) {
plate[j][endCol] = plate[j - 1][endCol];
min = Math.min(min, plate[j][endCol]);
}
plate[startRow + 1][endCol] = firstNum;
answer[i] = min;
}
return answer;
}
}
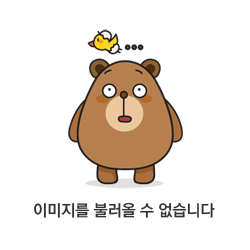
느낀 점
오류가 계속 떠서 이런 저런 방식으로 바꿔서 해본다고 열 엄청 받고, 시간을 엄청 많이 쓴 문제다. lv 2 난이도는 나한테 아직 쉽지 않다. 그래도 풀어서 다행
'알고리즘' 카테고리의 다른 글
자바 - 프로그래머스 / 광물 캐기 (0) | 2023.04.24 |
---|---|
자바 - 프로그래머스 / 연속된 부분 수열의 합 (0) | 2023.04.24 |
자바 - 프로그래머스 / 추억 점수 (0) | 2023.04.18 |
자바 - 프로그래머스 / 달리기 경주 (0) | 2023.04.17 |
자바 - 백준 20055 / 컨베이어 벨트 위의 로봇 (1) | 2023.04.13 |